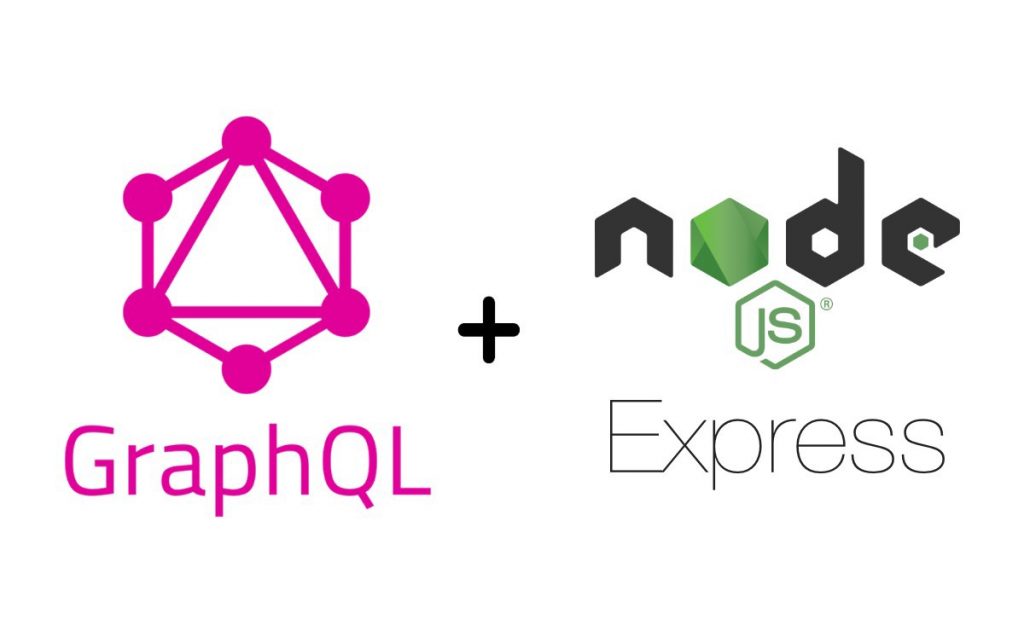
What is GraphQL?
GraphQL is a query language and an alternative for API requests. It uses server-side APIs to assist clients to get appropriate data. GraphQL, on the other hand, is more of syntax for creating queries, modifications, and schema.
What is Node.js?
Node.js is a JavaScript runtime environment that may be used to construct the backend and allows JavaScript to execute outside of the browser. It is highly preferred by Node.js development companies to speed up the backend development process using JavaScript and deliver projects on time.
GraphQL queries and mutations
GraphQL queries enable users to view and manipulate how data is received. Mutations are used to control how data is written on the server.
How to define schema?
Graphql schema is essential for any GraphqL server. It describes how your data is shaped, defining fields with types that are coming from your data source. It specifies which queries and mutations are available to the client.
// schema.graphql file
type Song {
title: String
author: Author
}
type Author {
name: String
songs: [Song]
}
Then, we must specify the query type to define the available queries:
type Query {
getSongs: [Song]
getAuthors: [Author]
}
But to keep it simple our schema will only have one single query type that will return a string.
type Query {
greeting: String
}
Any programming language can be used to create a GraphQL schema and build an interface accordingly. And there is also a server called Apollo server to execute GraphQL queries.
We will create a server in the index.js file.
To initialize the project will use npm commands and install Apollo-server as well.
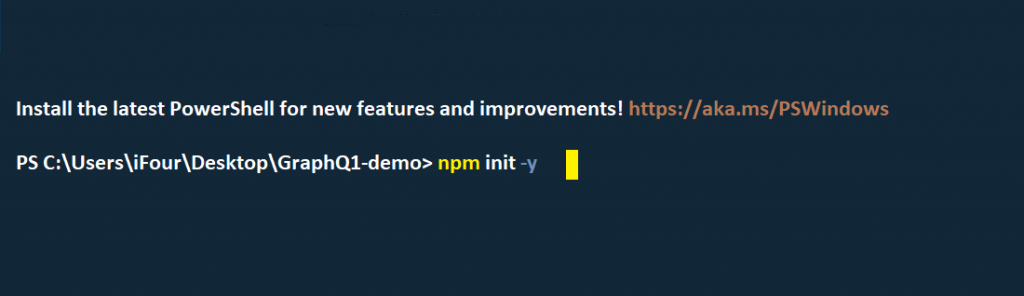
Fig-1.1 initiating Project
npm install apollo-server graphql
To parse the schema, we have to import the gql function from an Apollo-sever. And then create typedefs constants which is an abstract syntax from GraphQL code.
const { gql } = require('apollo-server');
const typeDefs = gql`
type Query {
greeting: String
}
`;
Add Resolver Function
A resolver function handles the data for each schema field. A third-party API or back-end database can send that data to the client. They just need to match the schema type.
const resolver = {
Query: {
greeting: () => 'Hii GraphQL world!',
},
};
A resolver function is a place where the query is written to manipulate data within a database.
Setting Up the Server
In the index.js to create a server, we need to create an ApolloServer object with Schema and resolver as a parameter.
const { ApolloServer, gql } = require('apollo-server')
const server = new ApolloServer({ typeDefs, resolver });
Then we start the server using listen() with the port specified in the parameters.
server
.listen({ port: 5000 })
.then((ServerInfo) => console.log(`Server running at ${ServerInfo.url}`));
This server info can further be restructured and we can get the URL directly.
server
.listen({ port: 5000 })
.then(({ url }) => console.log(`Server running at ${url}`));
//index.js
const { ApolloServer, gql } = require('apollo-server');
const typeDefs = gql`
type Query {
greeting: String
}
`;
const resolver = {
Query: {
greeting: () => 'Hii GraphQL world!',
},
};
const server = new ApolloServer({ typeDefs, resolver });
server
.listen({ port: 5000 })
.then(({ url }) => console.log(`Server running at ${url}`));
Now if we run the index file using node index.js, the server will be up and running. And we can see this in the output:
Server running at http://localhost:5000/
GraphQL vs REST: Key differences to consider
REST comes with no specification and has certainly no defined tools. REST focuses more on the durability of the API rather than the performance.
GraphQL is a query language defined to work with one endpoint through HTTP. And it also improves the performance of API.
Data Fetching
One of the significant things introduced in GraphQL is Data Fetching. In REST API to recover data, it requires making requests to numerous endpoints. With GraphQL, we only need to request a single endpoint to access data over the server.
Error Management
In REST error management is quite simple in comparison to GraphQL. To resolve the error all we need to do is to inspect the HTTP headers to get response information. While in GraphQL we always receive status code 200 with status OK.
Caching
REST is deployed over HTTP so it by default puts a cache into effect that can be used to get resources. Graphql does not come with a caching system, which is why users have to handle the cache on their own.
Advantages of Using GraphQL
Versioning
When restricted data control is returned from an API edge, any change can be considered a breaking change, and breaking variations necessitate an updated version. This is probably the most important reason why most APIs use versioning. If adding new features to an API necessitates using the most recent version, a trade-off must be made between publishing frequently and interpreting and retaining the API.
GraphQL in this situation only rebounds the data that needs current changes and that can be included with using types without occurring breaking change.
Performance Optimization
GraphQL is generally the smallest possible request, whereas REST usually defaults to the entirety. Although the REST API returns an elementary partial, GraphQL sends out more fragments by default.
Deprecation made easy
In GraphQL, any field can be deprecated. In that case, REST API works differently.
GraphQL makes it incredibly easy to watch over a particular field usage.
The disadvantage of using GraphQL
Authorization
The authorization issue is also an important consideration when working with GraphQL. Take GraphQL as an example of a domain-specific language. We could simply add a single layer between the data service and our clients. Authorization is a completely separate layer, and the language will not help you apply or use verification or authentication. You can, however, use GraphQL to connect the entry tokens between the clients and the plan. However, this is exactly the approach we take in REST.
Conclusion
Graphql provides a comprehensive description of data in API that helps end-users access the required data. While using GraphQL, HTTP is the most popular client-server protocol, owing to its widespread use. To put it simply, GraphQL serves the function that REST API cannot. In this blog, we learned about the significance of GraphQL, how it aids in server-side API execution, the benefits and downsides of GraphQL, and how it differs from REST API.
I am a Tech geek and blogger, a seasoned freelancer, and my hobby is to enlighten my views and skills that will helpful for new inductions of the industry.
Thanks again.